RS-232 Messaging
RS-232 messaging is used when Comm Port 2 > Port Type is set to RS-232.
An RS-232 message first sends a Start Bit '0'
, followed by seven bits of ASCII data with a parity bit to confirm the validity of the message, and terminated with a Stop Bit '1'
.
Often a script with several tasks is written in the gateway to send/receive custom packets via the RS-232 port when the task is executed.
For example, you can use the Start() statement at the top of the task so the task listens to the DyNet network for a particular message (e.g. a Preset message in a particular area), and then sends a custom string over the RS-232 port when the correct message is received.
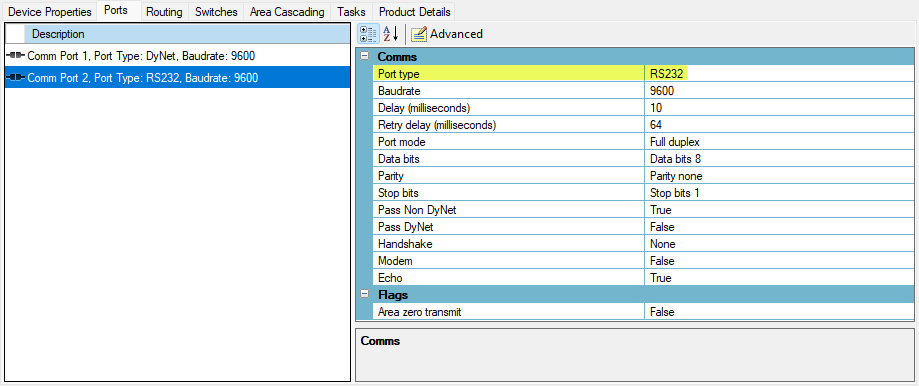
The same commands sent and received in Text over IP can be used over RS-232. |
Tasking
Tasking includes three command types that can be used with DyNet Text or RS-232:
-
Send2() transmits a custom (text or hex) string via Port 2.
-
SendP() transmits a custom message to an RS-232 port (or IP Port on an Ethernet gateway). You can send hex, decimal, or ASCII characters as raw data or from memory locations.
-
SendPF() is a more powerful version of the SendP() command, supporting the same instructions and more for sending text using the printf format. A single command can send values from up to 8 tilde memory locations.
Depending on the target device, the message may require a carriage return after the RS-232 message. this can be added by appending 0x0D to the Send2() command.
|
DyNet to RS-232
For this example, the task in the gateway listens for a Preset message to an area and then sends a command to a third-party projector to change the video input.
Name="DyNet to RS-232 example"
// DESCRIPTION
// This task listens to Area 32 dummy messages to trigger various commands towards a projector/beamer.
// Each preset represents a different command (See comments for details).
//MEMORY MAP:
// ~10 till ~16 DyNet receive buffer
Startup1()
{
Name="Start Task"
}
Task1()
{
Name="Projector control"
Start(0x1c,0x20,x,x,x,0x00,0xff) //Start on Area 32 message
COPY @0,~10,7 //Copy the incoming message to ram locations starting at Tilde mem 10
LDA ~13 //Load opcode byte from DyNet command into accumulator
CMP #0x0A //If opcode=0x0A (call preset 5) branch to P5A32
BRZ P5A32
CMP #0x0B //If opcode=0x0B (call preset 6) branch to P6A32
BRZ P6A32
CMP #0x0C //If opcode=0x0C (call preset 7) branch to P7A32
BRZ P7A32
CMP #0x0D //If opcode=0x0D (call preset 8) branch to P8A32
BRZ P8A32
Null
P5A32:
//Source = computer 1
Send2(0xBE,0xEF,0x03,0x06,0x00,0xFE,0xD2,0x01,0x00,0x00,0x20,0x00,0x00)
//Send serial command
Null
P6A32:
//Source = Computer 2
Send2(0xBE,0xEF,0x03,0x06,0x00,0x3E,0xD0,0x01,0x00,0x00,0x20,0x04,0x00)
//Send serial command
Null
P7A32:
//Source = Component
Send2(0xBE,0xEF,0x03,0x06,0x00,0xAE,0xD1,0x01,0x00,0x00,0x20,0x05,0x00)
//Send serial command
Null
P8A32:
//Source = HDMI
Send2(0xBE,0xEF,0x03,0x06,0x00,0x9E,0xD3,0x01,0x00,0x00,0x20,0x02,0x00)
//Send serial command
Null
}
RS-232 to DyNet
For this example, the task in the gateway listens for the volume level (0-100%) from an AV receiver and then sends a set channel level command (0-255) to the Dynalite system.
Name=" RS-232 to DyNet example"
M1: DyNet(0x1c,0x02,0xFF,0x80,0xff,0x64,0xff)
Send_Level:
#asm 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20,
21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40,
41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60,
61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80,
81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99, 100,
101; //x Don't care
Level1:
#asm 255, 254, 252, 249, 247, 244, 242, 239, 237, 234, 232, 229, 227, 224, 221,
219, 216, 214, 211, 209, 206, 204, 201, 199, 196, 194, 191, 188, 186, 183,
181, 178, 176, 173, 171, 168, 166, 163, 161, 158, 155, 153, 150, 148, 145,
143, 140, 138, 135, 133, 130, 127, 125, 122, 120, 117, 115, 112, 110, 107,
105, 102, 100, 97, 94, 92, 89, 87, 84, 82, 79, 77, 74, 72, 69, 67, 64, 61,
59, 56, 54, 51, 49, 46, 44, 41, 39, 36, 34, 31, 28, 26, 23, 21, 18, 16, 13,
11, 8, 6, 3, 0;
Startup1()
{
Name="Start Task"
LDX #0
}
Task1()
{
Name="RS-232 to DyNet"
Startx(x,0x25) //Listen to a Value between "0%-100%" from AV receiver - Send Corresponding 0-100% ChannelLevel
Copy @0,~0,2 //Copy incoming 2 Bytes from AV
LDX #0 //Initialise the X register
Loop1:
LDA Send_Level,x //Load value from Array in reference to the X register value
CMP ~0 //Compare this Value with the Value received from the AV (a Number between 0-100
BRZ Send_it //If matching assemble and send the channelLevel message
INCX //If not a Match, increment the x register Value
CMPX #103 //make sure X register is less than 103
BRZ END //If 103 or greater, Send a No Match message
BRA Loop1 //Else Branch back to Loop1
Send_it:
Copy M1,~10,7 //Copy default ChannelLevel message
LDA Level1,x //Load the Accumulator with the value in "Level1" Array that corresponds with the x register value
STA ~12 //store this Value in Data1 (level byte)
TX ~10,2,7 //transmit the corresponding ChannelLevel message
Null
END:
Dynet(0x6c,"NoMtch") //Incase X register Value is greater or equal to 103
}
ASCII Map
Char | Dec | Hex | Keyboard Escape | Escape String | Char | Dec | Hex | Keyboard Escape | Escape String |
---|---|---|---|---|---|---|---|---|---|
(nul) |
0 |
0x00 |
(dle) |
16 |
0x10 |
Ctrl+P |
|||
(soh) |
1 |
0x01 |
Ctrl+A |
(dc1) |
17 |
0x11 |
Ctrl+Q |
||
(stx) |
2 |
0x02 |
Ctrl+B |
(dc2) |
18 |
0x12 |
Ctrl+R |
||
(etx) |
3 |
0x03 |
Ctrl+C |
(dc3) |
19 |
0x13 |
Ctrl+S |
||
(eot) |
4 |
0x04 |
Ctrl+D |
(dc4) |
20 |
0x14 |
Ctrl+T |
||
(enq) |
5 |
0x05 |
Ctrl+E |
(nak) |
21 |
0x15 |
Ctrl+U |
||
(ack) |
6 |
0x06 |
Ctrl+F |
(syn) |
22 |
0x16 |
Ctrl+V |
||
(bel) |
7 |
0x07 |
Ctrl+G |
(etb) |
23 |
0x17 |
Ctrl+W |
||
(bs) |
8 |
0x08 |
Ctrl+H |
(can) |
24 |
0x18 |
Ctrl+X |
||
(ht) |
9 |
0x09 |
Ctrl+I |
(em) |
25 |
0x19 |
Ctrl+Y |
||
(nl) |
10 |
0x0A |
Ctrl+J |
\n |
(sub) |
26 |
0x1A |
Ctrl+Z |
|
(vt) |
11 |
0x0B |
Ctrl+K |
(esc) |
27 |
0x1B |
Ctrl |
||
(np) |
12 |
0x0C |
Ctrl+L |
(fs) |
28 |
0x1C |
Ctrl |
||
(cr) |
13 |
0x0D |
Ctrl+M |
\r |
(gs) |
29 |
0x1D |
Ctrl |
|
(so) |
14 |
0x0E |
Ctrl+N |
(rs) |
30 |
0x1E |
Ctrl |
||
(si) |
15 |
0x0F |
Ctrl+O |
(us) |
31 |
0x1F |
Ctrl |
Char | Dec | Hex | Char | Dec | Hex | Char | Dec | Hex | Char | Dec | Hex | Char | Dec | Hex | Char | Dec | Hex |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
(sp) |
32 |
0x20 |
0 |
48 |
0x30 |
@ |
64 |
0x40 |
P |
80 |
0x50 |
` |
96 |
0x60 |
p |
112 |
0x70 |
! |
33 |
0x21 |
1 |
49 |
0x31 |
A |
65 |
0x41 |
Q |
81 |
0x51 |
a |
97 |
0x61 |
q |
113 |
0x71 |
" |
34 |
0x22 |
2 |
50 |
0x32 |
B |
66 |
0x42 |
R |
82 |
0x52 |
b |
98 |
0x62 |
r |
114 |
0x72 |
# |
35 |
0x23 |
3 |
51 |
0x33 |
C |
67 |
0x43 |
S |
83 |
0x53 |
c |
99 |
0x63 |
s |
115 |
0x73 |
$ |
36 |
0x24 |
4 |
52 |
0x34 |
D |
68 |
0x44 |
T |
84 |
0x54 |
d |
100 |
0x64 |
t |
116 |
0x74 |
% |
37 |
0x25 |
5 |
53 |
0x35 |
E |
69 |
0x45 |
U |
85 |
0x55 |
e |
101 |
0x65 |
u |
117 |
0x75 |
& |
38 |
0x26 |
6 |
54 |
0x36 |
F |
70 |
0x46 |
V |
86 |
0x56 |
f |
102 |
0x66 |
v |
118 |
0x76 |
' |
39 |
0x27 |
7 |
55 |
0x37 |
G |
71 |
0x47 |
W |
87 |
0x57 |
g |
103 |
0x67 |
w |
119 |
0x77 |
( |
40 |
0x28 |
8 |
56 |
0x38 |
H |
72 |
0x48 |
X |
88 |
0x58 |
h |
104 |
0x68 |
x |
120 |
0x78 |
) |
41 |
0x29 |
9 |
57 |
0x39 |
I |
73 |
0x49 |
Y |
89 |
0x59 |
i |
105 |
0x69 |
y |
121 |
0x79 |
* |
42 |
0x2A |
: |
58 |
0x3A |
J |
74 |
0x4A |
Z |
90 |
0x5A |
j |
106 |
0x6A |
z |
122 |
0x7A |
+ |
43 |
0x2B |
; |
59 |
0x3B |
K |
75 |
0x4B |
[ |
91 |
0x5B |
k |
107 |
0x6B |
{ |
123 |
0x7B |
, |
44 |
0x2C |
< |
60 |
0x3C |
L |
76 |
0x4C |
\ |
92 |
0x5C |
l |
108 |
0x6C |
| |
124 |
0x7C |
- |
45 |
0x2D |
= |
61 |
0x3D |
M |
77 |
0x4D |
] |
93 |
0x5D |
m |
109 |
0x6D |
} |
125 |
0x7D |
. |
46 |
0x2E |
> |
62 |
0x3E |
N |
78 |
0x4E |
^ |
94 |
0x5E |
n |
110 |
0x6E |
~ |
126 |
0x7E |
/ |
47 |
0x2F |
? |
63 |
0x3F |
O |
79 |
0x4F |
_ |
95 |
0x5F |
o |
111 |
0x6F |
(del) |
127 |
0x7F |